KitchenDraw Plugins
A KitchenDraw plugin is a file which contains functions extending the basic
functionalities of the KitchenDraw software. A function belonging to a plugin
(that is correctly registered) is called by the KitchenDraw application when
the corresponding event is fired. For example, OnAppStartAfter
function
is called automatically each time KitchenDraw launches.
Though the plugin can be implemented in several ways:
- As a .NET assembly,
- As an ActiveX .DLL,
- As an ordinary Windows .DLL,
- As a javascript file (.JS).
this documentation focuses primarily on developing plugins with the .NET technology. Examples in this documentation are given in C#, but you can use any other language to develop a plugin as well.
Developing Plugins
The code of a very basic KitchenDraw plugin, Sample.dll
, can look like this:
using KD.SDK2;
using System.Windows.Forms; // to use MessageBox
namespace Sample
{
public class Plugin
{
bool OnAppStartAfter(int iCallParams)
{
var appli = new Appli();
MessageBox.Show(string.Format("Your account number is {0}.",
appli.AccountNumber));
MessageBox.Show(string.Format("Current language is {0}.",
appli.LanguageCode));
return true;
}
}
}
A valid plugin must follow these conventions:
- The main class of your plugin must be called
Plugin
. The class itself and its parameterless constructor must be declaredpublic
. - The namespace of the
Plugin
class must have the same name as the generated assembly, excludion the .DLL extension (e.g. for the Sample.dll assembly the namespace shoule beSample
, while for the Acme.Wizard.dll assembly namespace should beAcme.Wizard
).
In addition, these are the general recommendations for the plugin development:
- Preserve the case for the .DLL filenames, namespaces and classes.
- Keep
Plugin
class constructor as clean as possible. UseOnPluginLoad
andOnPluginUnload
events for the installation and cleaning phases of your plugins. For example, you can add a custom menu item inOnPluginLoad
and remove it inOnPluginUnload
. - Add to your project a reference to KD.SDK2.DLL to be able to benefit from the IntelliSense features.
- The plugins you create don't have to be registered (e.g. with regasm).
- To be executed, your plugin should be registered in KitchenDraw. See later how to do it.
- Test your plugins with the highest UAC restrictions level before deployment.
- It is highly recommended to numerically sign your plugins prior to any deployment.
Accessing KitchenDraw
All the plugins get current KitchenDraw session by default. Just create an instance of the Appli class - and you are already connected to the current application context.
bool OnPluginLoad(int unused)
{
var appli = new KD.SDK2.Appli();
// The appli class is connected to the current KitchenDraw session by
// default, thus you can use your appli instance without any additional
// setup.
MessageBox.Show(appli.LanguageCode);
return true;
}
Plugin Events
A certain number of events occurring during the use of the KitchenDraw software
allow launching the execution of their corresponding event handlers located in
plugins that are loaded into memory. These event handlers are called plugin
functions in the documentation, and are merely a public methods of your Plugin
class. For example, OnSceneInformationBefore
plugin function is
executed when the user launches the "Scene|Information" command, just before
the corresponding dialog box appears.
You can learn more about plugin events here.
Global And Embedded Plugins
The plugins described previously whatever they are .NET, ActiveX, ordinary .DLL, JavaScript plugins are plugins that could be qualified as global plugins because they run independently of the catalogs used. This can be a problem for certain functions touching the objects of the scene.
For example, an OnObjectPlaceAfter
plugin function belonging to a global
plugin will be called (executed) after any object is placed into the scene
whatever its catalog of origin. However, it is often suitable that this kind of
function is called only for certain well identified objects (e.g. objects from
a given catalog or even objects having a particular reference).
The useless calls to plugin functions reduce the system performances. Moreover, a global plugin is separated from a catalog even if it is dedicated to managin only some objects of the catalog. This could lead to version inconsistency risks and makes it more difficult to deploy both the catalog and the plugin at the same time.
That's why embedded plugins technology was created. Embedded are the same plugins as global, they are placed inside catalog resources.
The differences between these embedded plugins and global plugins are the following:
- Additional events related to identified objects of the scene and belonging to the catalog can be managed: the placement, the deletion, the modification, the change of position, orientation or dimension of an object, the price calculation as well as the opening of the contextual menu when an object is active (allowing the addition of a menu item). Caution: the removal of a component triggers an OnChange modification event to the component object as well as to the "parent" object.
- The association between a plugin function and an event can be done block per block in the block scripts.
To learn more on embedded plugins please refer to this article.
Installing Your Plugins
To be taken into account by the KitchenDraw software, your plugin must be registered in KitchenDraw.
For global plugins, this is done by adding an entry into the SPACE.INI file. There are two methods can be employed as you prefer:
- You can declare your plugin in every section dedicated to particular event. This method allows you to register only some of your plugin functions, and it allows to control the plugin execution order for each event as well.
[OnAppStartAfter]
Plugins\YourPlugin.dll=
[OnFileOpenBefore]
Plugins\YourPlugin.dll=
- Or you can declare your plugin in the [Plugins] section. At startup, the KitchenDraw application will analyze the contents of your plugin and register all the events that are declared in it.
[Plugins]
Plugins\YourPlugin.dll=1
A SPACE.INI entry for a plugin entry consists of plugin path, equal sign, and load flag. Plugins which have the flag set to 1 in their entries will be loaded and executed by the KitchenDraw software automatically. Plugins which are registered with the flag set to 0 will be registered but not loaded into the application automatically. You can load them eigher via the Plugin Manager tool or programmatically from another plugin. If you omit load flag, it will be considered as set to 1:
Plugins\YourPlugin.dll=
is the same as:
Plugins\YourPlugin.dll=1
Your plugin can be registered using either absolute or relative path. .NET, ActiveX and ordinary .DLL plugin paths are relative to the KitchenDraw application directory. JavaScript plugin paths are relative to Scripts subdirectory of the KitchenDraw application directory. The filename in the path is case sensitive since it is used to determine the namespace of a plugin.
Embedded plugins are loaded into memory automatically without necessity of additional registration. However, you can disable their loading by creating an entry in the SPACE.INI file in the following format:
[PluginsFromCatalog]
<catalog filename>|<plugin filename>=0
example.cat|EmbeddedPlugin.dll=0
Please be aware that KitchenDraw plugins aren't loaded if the account has no more hours of use and there is no dongle connected.
The Plugin Manager Tool
The KitchenDraw software is bundled with the "Plugin Manager" tool which can be activated via the ? > Service > Plugin Manager menu.
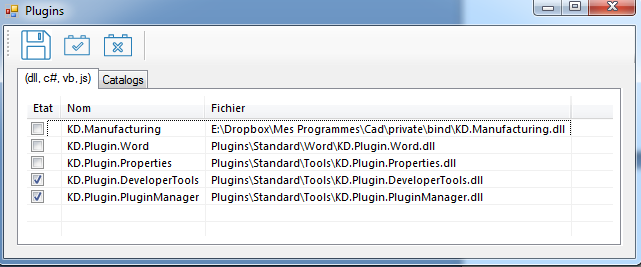
As its name indicates, the Plugin Manager manages the loaded/unloaded status of the KitchenDraw Plugins. It deals with both global and embedded plugins.
The "DLL, c#, vb, js" tab of the Plugin Manager lists the global plugins, while the "Catalogs" tab lists the embedded plugins from catalogs.
The Plugin Manager allows registering plugins and defining their activated or unactivated status and eventually saving the configuration in the [Plugins] and [PluginsFromCatalog] sections of the SPACE.INI file.
Debugging Your Plugins
Debugging of global plugins can be performed as usual. Just register your plugin in the KitchenDraw installation, and set CP.EXE or KD_APP.EXE as an external program to run for your plugin project debugging.
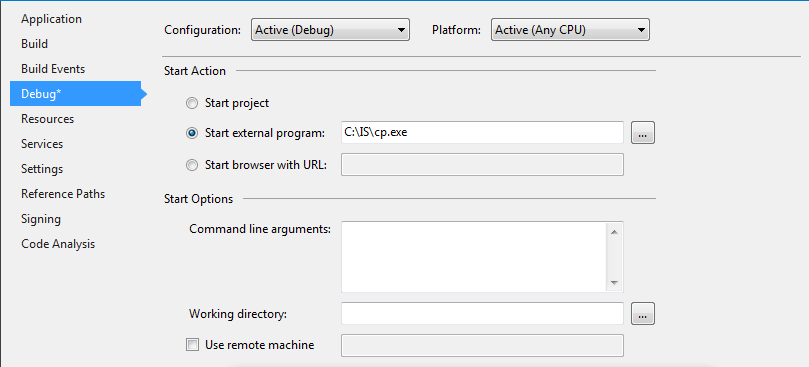
Debugging of embedded plugins can be performed by attaching to a running KitchenDraw process.
Start KitchenDraw application
Start Visual Studio and choose Debug > Attach to process in its main menu.
Choose your running KitchenDraw instance in the "Attach To Process" dialog box.
You can now debug as usual.
The procedure above is appropriate for many cases but not for the debugging of plugin functions which run during KitchenDraw startup since they are usually fired before you attach to a running process. To work around this problem, you can place Debugger.Launch call to your code like this:
public bool OnPluginLoad(int unused)
{
// ...
System.Diagnostics.Debugger.Launch();
// ...
}
This will show "Just-In-Time debugger" system dialog window, allowing you to attach a debugger and to debug startup method beginning from the line normally.
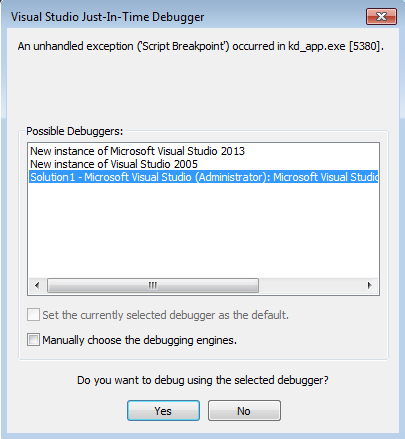